Model Deployment with Streamlit
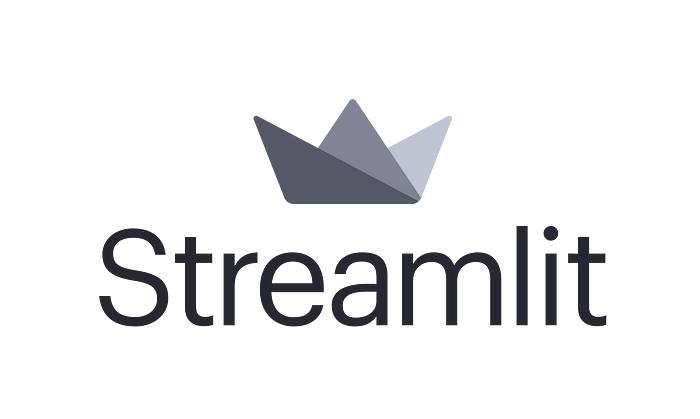
Streamlit is a open source python library for building fast, performant and beautiful data apps. It is a great tool to develop interactive apps quickly for demonstrating a solution.
In this story, we going to talk about how to deploy car price prediction model with using streamlit library.
Creating Streamlit App
First off all you need to install Python 3.6+ in your system in order to work streamlit properly.
1-) Install streamlit using PIP as you see below:
$ pip install streamlit
We will check our car price prediction model before create app.
This is the final version of our auto dataframe, we dropped useless columns removed outliers, cleaned the data, implemented get_dummies function to categorical columns, and finally obtained this dataset you see below. We can train the dataset now.

We used Random Forest and Xgboost regressor to train model.
from xgboost import XGBRegressorxgb_model = XGBRegressor(n_estimators = 1500, learning_rate = 0.05, max_depth= None, subsample =0.8)
xgb_model.fit(X_train, y_train,
early_stopping_rounds=10,
eval_set=[(X_test, y_test)],
verbose=True)y_pred = xgb_model.predict(X_test)r2_score: 0.9113619721765008
mae: 1411.5892836674725
mse: 4628539.5361547815
rmse: 2151.4040848140967
We obtained 0.91 accuracy score from Xgboost model.
rf_model=RandomForestClassifier().fit(X_train, y_train)y_pred = rf_model.predict(X_test)
confusion_matrix(y_test, y_pred)r2_score: 0.8976659819862614
mae: 1448.715421300877
mse: 5343722.777918033
rmse: 2311.6493630994373
We obtained 0.89 accuracy score from the Random forest model. We will use both of them in our application for variety.
After that, we saved our model in pickle format to callback when we create the app.
model_xgb = pickle.dump(xgb_model,open('xgb_model', 'wb'))
model_rf = pickle.dump(rf_model, open("rf_model","wb")
Clients who will use this application need to enter information like this below.
my_dict = {
"hp": 200,
"age": 1,
"km": 50000,
"model": 'A3',
"make": "Opel"
}df=pd.DataFrame.from_dict([my_dict])#returned this dictionary to dataframe to do prediction
However in order to do prediction we should return values to get_dummies format as you see below.

We can fix this using reindex and get_dummies methods. However we need columns name list we can handle this with columns method.
columns= X.columnsdf = pd.get_dummies(df).reindex(columns=columns, fill_value=0)
We append all columns in the user input dataframe and reindex method just received relevant user inputs , and return other rows from nan to zero with fill_value=0 parameter.
Creating a Car Price Prediction App
It is a necessary to create an app.py file to run our application. And bring together files we created before in a folder. Model files and final version of our data frame etc.
If you want to examine this application you can visit my GitHub repository.
# first we imported libraries we needimport streamlit as st
import pickle
import pandas as pd# Create a app title with title methodst.title('Car Price Prediction')# We called back our models created beforemodel1 =pickle.load(open("xgb_model","rb"))
model2= pickle.load(open("rf_model","rb"))# We use selectbox method and append our models to give a choice clientsmodels = st.selectbox("Select Model",("Random Forest","XGBoost") )# And specified a condition if users select Random forest use random forest model else use Xgboost model.if models == "Random Forest":
model = model2
else :
model = model1# We created selectbox for categorical columns and used slider numerical values ,specified range and step age = st.selectbox("What is the age of your car?",(1,2,3))
hp = st.slider("What is the horsepower of your car",60,200,step=5)
km=st.slider("What is the km of your car?",0,100000,step=500)
car_model=st.selectbox("Select model of your car", ('A1', 'A2', 'A3','Astra','Clio','Corsa','Espace','Insignia'))# in order to recieved client inputs appended these inputs (created above) into dictionary as we mentioned before. And We returned into dataframe.my_dict = {
"age": age,
"hp": hp,
"km": km,
"model": car_model
}df = pd.DataFrame.from_dict([my_dict])# And appended column names into column list. We need columns to use with reindex method as we mentioned before.df = pd.get_dummies(df).reindex(columns=columns, fill_value=0)# We append all columns in the user input dataframe and reindex method just received relevant user inputs , and return other columns from nan to zero with fill_value=0 parameter.# And now we can predictprediction = model.predict(df)# Success method demonstrate our prediction in a green squarest.success("The estimated price of your car is €{}. ".format(int(prediction[0])))
And now all we have to do run “streamlit run app.py” command in the anaconda terminal. But first, you need to go to the path where your files are with using the cd command.

And final version of the our model.

Thank you for reading…